Strong reference cycle experiments, on iOS 14.4 (XCode 12.4).
A good official article on Automatic Reference Counting (ARC) in Swift.
A holding a reference to B, and B holding a reference to A
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
import UIKit
class ViewController: UIViewController {
var b = B()
override func viewDidLoad() {
super.viewDidLoad()
let a = A(b: self.b)
self.b.a = a
}
}
class A {
let b: B
init(b: B) {
self.b = b
}
}
class B {
var a: A?
init() {
self.a = nil
}
}
|
From the memory graph, it’s pretty clear that object A and B have strong reference cycle.
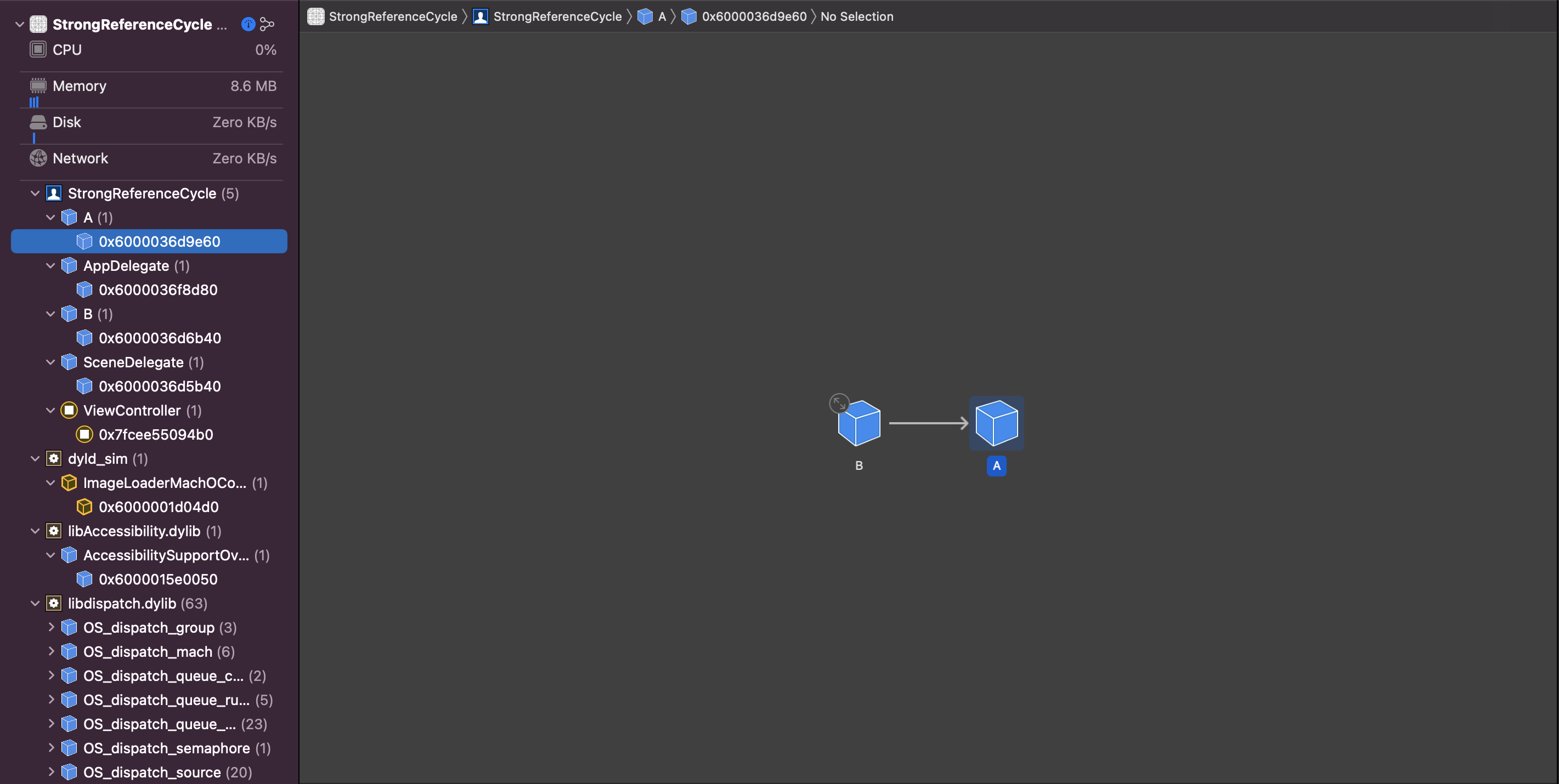
The lazy var closure
Will have strong reference cycle
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let heading = HTMLElement(name: "h1")
print(heading.asHTML())
}
}
class HTMLElement {
let name: String
let text: String?
lazy var asHTML: () -> String = {
if let text = self.text {
return "<\(self.name)>\(text)</\(self.name)>"
} else {
return "<\(self.name) />"
}
}
init(name: String, text: String? = nil) {
self.name = name
self.text = text
print("\(name) is being initialized")
}
deinit {
print("\(name) is being deinitialized")
}
}
|
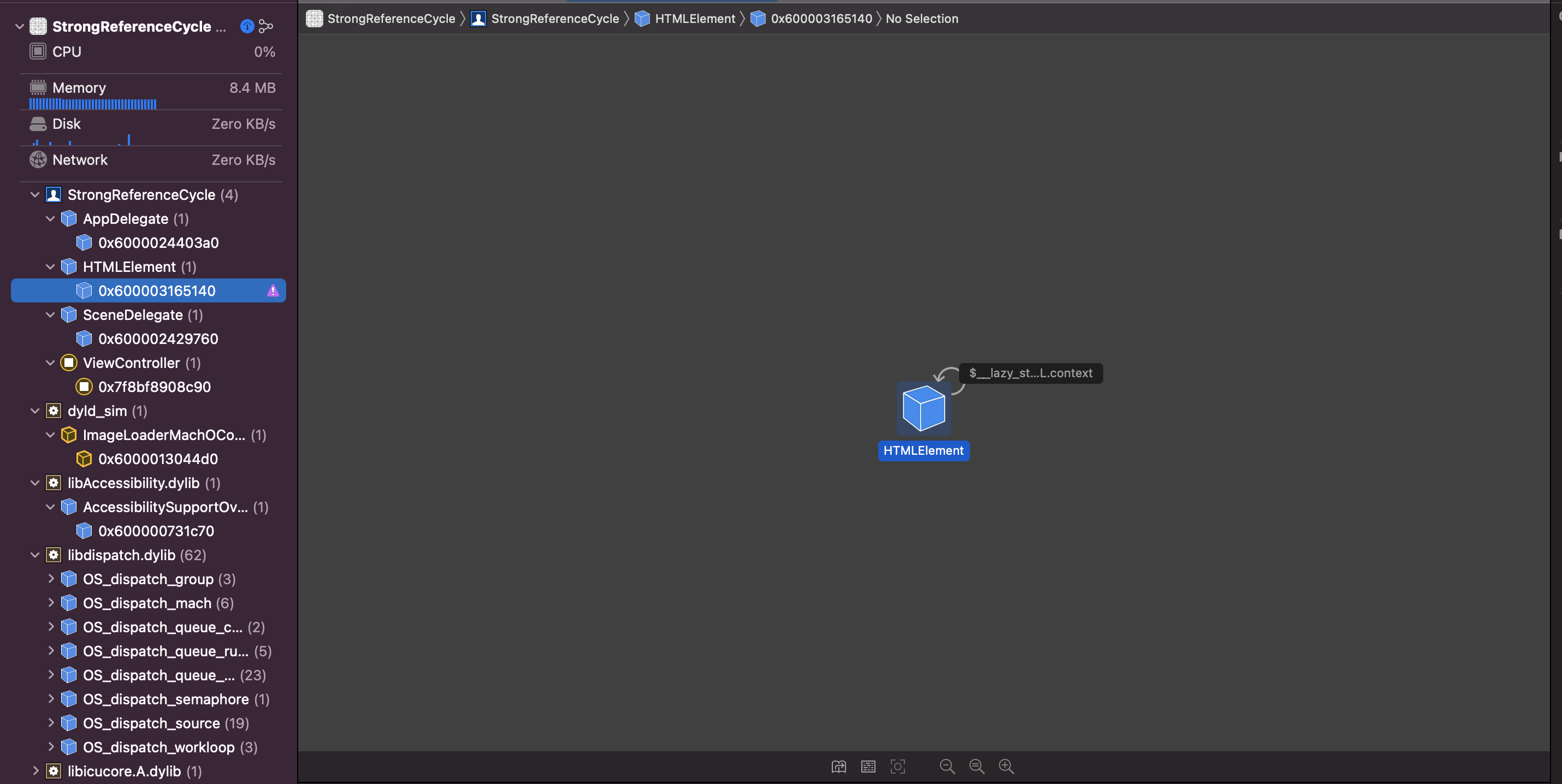
Will NOT have strong reference cycle
But if you change lazy var asHTML: () -> String
to lazy var asHTML: String
and the code accordingly, the strong reference cycle won’t happen!
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let heading = HTMLElement(name: "h1")
print(heading.asHTML)
}
}
class HTMLElement {
let name: String
let text: String?
lazy var asHTML: String = {
if let text = self.text {
return "<\(self.name)>\(text)</\(self.name)>"
} else {
return "<\(self.name) />"
}
}()
init(name: String, text: String? = nil) {
self.name = name
self.text = text
print("\(name) is being initialized")
}
deinit {
print("\(name) is being deinitialized")
}
}
|